In this tutorial I would like to share with you a ready to use example how you can sync WooCommerce products and variations inventory with a CSV file automatically.
Our CSV file is going to look like this:
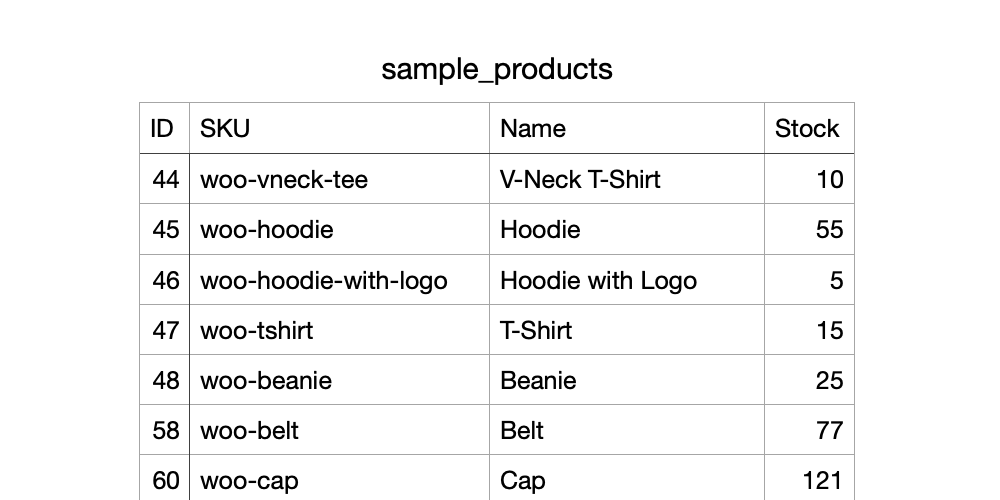
To make this tutorial a little bit simpler we are going to put the file in the WordPress uploads folder, but for sure you can create a WooCommerce settings page where you can upload it.
You might also be interested in a plugin that allows to sync product inventory of products with the same SKU between different WooCommerce stores.
Below is the function that does all the stuff:
function wpdev_sync_product_stock_from_csv( $filename = 'sample_products.csv' ) {
$upload_dir = wp_upload_dir();
// so the file is always in wp-content/uploads dir
$filename = "{$upload_dir[ 'basedir' ]}/{$filename}";
if( ! file_exists( $filename ) ) {
return false;
}
$file = fopen( $filename, 'r' );
// it is not necessary but we can format the CSV result into a readable array
$csv_products = array();
while( false !== ( $result = fgetcsv( $file, 100, ";" ) ) ) {
$csv_products[] = array(
'sku' => $result[1],
'stock_qutantity' => $result[3]
);
}
array_shift( $csv_products ); // remove heading of the product table
// now we have
// Array( sku => , stock_qutantity => )
// it is time to loop through the products and update their stock quantities
foreach( $csv_products as $csv_product ) {
// in case stock quantity value is empty in CSV file
if( empty( $csv_product[ 'stock_qutantity' ] ) || ! $csv_product[ 'stock_qutantity' ] ) {
continue;
}
// let's try to get a product from SKU
$product_id = wc_get_product_id_by_sku( $csv_product[ 'sku' ] );
if( ! $product_id ) {
continue;
}
// let's try to get a product object next
$product = wc_get_product( $product_id );
if( ! $product ) {
continue;
}
$product->set_manage_stock( true );
$product->set_stock_quantity( $csv_product[ 'stock_qutantity' ] );
$product->save();
}
}
Let’s take a closer look at the code:
- In order to parse a CSV file we are using fgetcsv() PHP function and its the second parameter which is 100 at this example contains the maximum length of the row, you can set it to 0 or null but it may slow down the function a little.
- Each time we use fgetcsv() in a loop it makes a row increment, which could be increadibly helpful when you’re working with large CSV files. So you can parse just a specific amount of rows into $csv_products and do not use wc_get_product() and set_stock_quantity() let’s say thousands times in a single loop.
- I used array_shift() on line 21 because the first row is the table heading which we don’t obviously need here.
- Using method $product->set_manage_stock() is not a necessary thing here but might be useful if for some products listed in CSV file with stock managemant is not enabled.
- Also functions wc_get_product_id_by_sku() and wc_get_product() work great for both products and product variations.
Below is the code example how you can make the CSV file to parsed automatically every hour.
add_action( 'wpdev_doing_csv_sync', 'wpdev_sync_product_stock_from_csv' );
if( ! wp_next_scheduled( 'wpdev_doing_csv_sync' ) ) { wp_schedule_event( time(), 'hourly', 'wpdev_doing_csv_sync' ); }